Security Risks of Exposed Directories in Android FileProvider
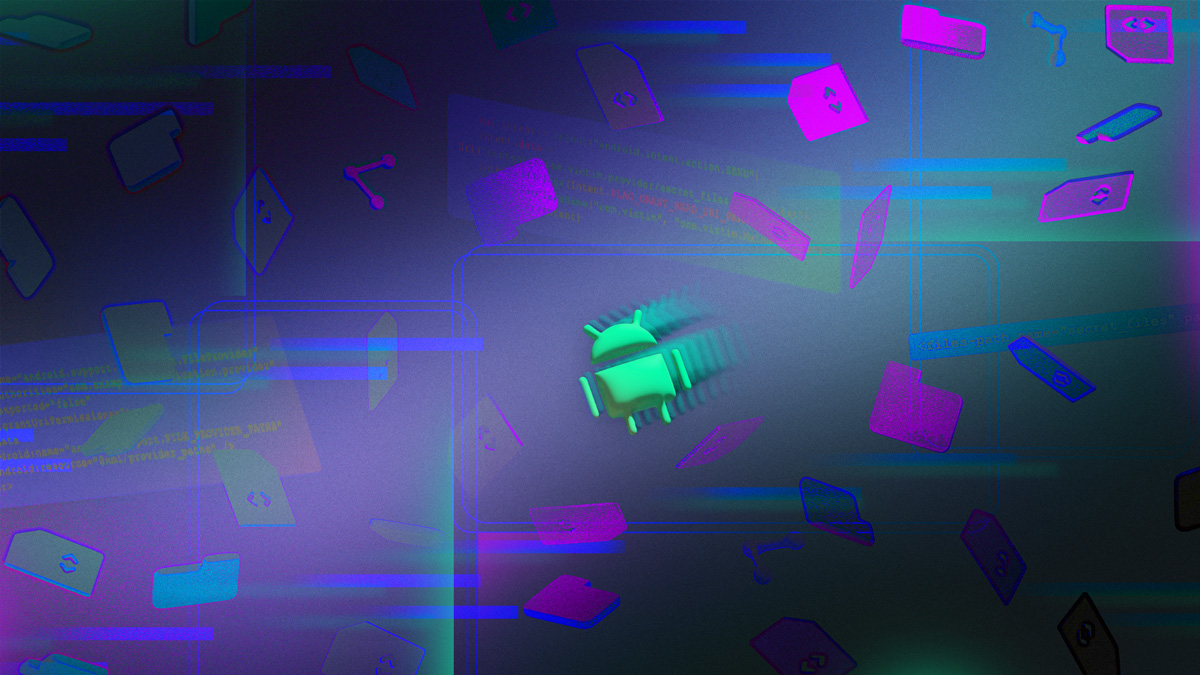
When it comes to safely sharing content with third-party Android apps, ContentProviders are meant to be used. Using those, you can generate secure URIs for all kinds of data ensuring that only authorized third-party applications can access the shared resources, making it a structured and secure way to share data.
In this article we will discuss the Android FileProvider, a specialized subclass of ContentProvider that provides secure sharing of app’s internal files, allowing developers to grant temporary read and write file access.
Despite being a secure mechanism for file sharing, the FileProvider needs to be properly configured to prevent encountering security-related incidents. Exploitation of a misconfigured FileProvider could lead to privacy issues, theft of sensitive data and unauthorized disclosure of private information, which are all significant security threats to the application. Such scenarios are most likely to happen when an application configures FileProvider with overly permissive file paths, shares files with other apps or inadequately validates incoming Intents. This security issue is also mentioned in the OWASP Top Ten Mobile Risks 2023 as a part of the Security Misconfiguration section.
In this blog post, we'll delve into the risks associated with improperly exposed directories through FileProvider and explore best practices to enhance Android app security.
Android FileProvider basics:
Let’s begin by getting a basic understanding of a FileProvider configuration in an Android app. Setting up a FileProvider involves several steps, which will be broken down one by one.
- First, a
<provider>
element is included in the app's AndroidManifest.xml file. It contains a number of attributes, which define the FileProvider configuration:android:name
is the name of the FileProvider implementation class used in the application.android:authorities
specifies a unique identifier for a ContentProvider, enabling other apps to interact with it using content URIs.android:exported
indicates whether the ContentProvider can be accessed by other apps and is recommended to be set tofalse
.android:grantUriPermissions
allows granting temporary access to files.
- The
<meta-data>
element points to an XML file that contains paths which the FileProvider will be able to share with other applications via content URIs.
<provider
android:name="android.support.v4.content.FileProvider"
android:authorities="com.example.myapplication.provider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/provider_paths" />
</provider>
- The file paths that are intended to be shared via FileProvider need to be specified in the file referenced in
<meta-data>
. These paths should point to the directories or specific files that will be shared. The file can contain elements like<files-path>
,<exported-path>
,<root-path>
, etc., each of them structured in the following way:
<files-path name="my_images" path="images/"/>
- Next, in the application's code, FileProvider is used to generate content URIs for the files to be shared. To share a file, an Intent including the corresponding content URI should be constructed and activated.
String uriToSend = FileProvider.getUriForFile(this, "com.example.myapplication.provider", fileToShare);
intent.setData(uriToSend);
...
startActivity(intent);
FileProvider configuration risks
With the configuration presented above, FileProvider can only give access to files located in the images/ folder. So, even if this application grants permissions to apps that should not have access to certain files or does not carefully manage the way URIs are sent, in the worst case scenario only the images/ directory may be exposed to an attacker.
But let’s take a look at what issues might arise if the developer configures access paths like this:
<files-path name="my_files" path="."/>
Here the entire folder with the app's internal files is being set as a FileProvider path. Yet again, if the application is not careful about how it sends URIs and handles incoming data, basically any internal files may be exposed to the attackers.
Similar applies to the usage of<root-path>
element in the configuration.<root-path>
directly links to the device's root directory. So, using it in the configuration means allowing unrestricted access to files and folders, for instance to the app's isolated sandbox environment, which significantly expands an attack surface.
<root-path name="my_root" path="/"/>
Keep in mind that even if the sending app's FileProvider configuration includes.
and allows sharing files from the current directory, the malicious receiver app is not allowed to access a file that it has not been explicitly granted access to. The Android permission system enforces these restrictions.
However, application’s code may contain other security gaps that can allow attackers to get access to the files that are not directly shared by the application. Therefore, it is still considered potentially vulnerable because as such cases may lead to unintentional data exposure or tampering.
EXAMPLE:Now we will take a look at an example of such risk.
Let’s say we have two apps: victim and attacker.
The victim app configures and uses FileProvider to share files with other applications. The FileProvider’s file paths are set like this:
<files-path name="secret_files" path="."/>
As mentioned above, such configuration alone does not lead to data leaks. So, we will assume the victim app contains a second vulnerability. Namely, an intent being sent back without proper validation.
Android intents serve as a communication tool in Android development, facilitating interactions between different components. They are for example used to launch activities or share information between apps or components of the same app. Moreover, what’s interesting in our case, file access can be controlled via intent flags.
So, let’s say we have this code in the victim app:
val receivedIntent = intent // Receiving an external intent
val data = intent.data // Getting data from the intent
this.setResult(-1, receivedIntent) // Sending an intent back
Here the app receives an external Intent, gets information from it and sends it back to the sender. Note how no validation of the Intent’s contents is done. This allows an attacker to exploit the issue in the FileProvider above.
To exploit this vulnerability the attacker app can send an Intent containing the file URI it wants to extract, as well as the required permission to the victim app:
val intent = Intent("android.intent.action.SEND")
intent.data = Uri("content://com.victim.provider/secret_files/secrets.txt")
intent.addFlags(Intent.FLAG_GRANT_READ_URI_PERMISSION)
intent.setClassName("com.victim", "com.victim.MainActivity")
sendIntent(intent)
Since the victim app does not validate or change the content of this Intent, it sends it back with the read permission of the secrets file.
By reverse engineering the victim app, attackers can take a look at all the configuration files and construct valid URIs to access the files of the victim application attackers are interested in.
And as FileProvider is configured with .
as its path - all the files under .
directory become accessible for the attackers.
Using this attack, an attacker app installed on the same device as the vulnerable app, can extract potentially sensitive data from the victim’s internal storage.
5 best practices for Android FileProviders
To mitigate the risks, Android developers should follow best practices when using FileProvider:
- Avoid using overly broad paths like
.
or/
, aim at using more specific paths. The FileProvider configuration file allows developers to specify several entries of elements like<files-path>
, which comes in handy in this case. - Create scoped directories within your app's data directory to store the files you want to share. This helps maintain a well-structured, limited-access environment.
- Validate the URIs coming from external sources and check that they are not pointing to your application’s local files which you did not explicitly grant access to.
- When sending Intents, grant only necessary minimal access permissions, i.e. do not grant write permission if it is not needed.
Conclusion
To sum up, Android’s FileProvider is a powerful tool for sharing files between apps, while maintaining security and privacy. However, Android app developers need to ensure that they configure FileProvider correctly to prevent risks that involve unauthorized access to sensitive files and the potential exposure of confidential information by the attackers.
By adhering to best practices and regularly reviewing configurations, one can maintain the security and integrity of their app.